import gc
gc.disable()
Garbage Collection
Garbage collection is a form of automatic memory management. The garbage collector, or just collector, attempts to reclaim garbage, or memory occupied by objects that are no longer in use by the program.
Garbage collection is important because it helps to prevent memory leaks. A memory leak occurs when a program allocates memory but does not deallocate it, leading to memory exhaustion.
Garbage collection is also important because it helps to prevent memory fragmentation. Memory fragmentation occurs when the memory is divided into small, non-contiguous blocks, leading to inefficient memory usage.
In the context of data science and pandas, garbage collection is important because data science projects often involve working with large datasets, which can consume a significant amount of memory. If memory is not properly managed, it can lead to performance issues and crashes.
In Python, garbage collection is handled by the gc
module. The gc
module provides an interface to the optional garbage collector. It provides the ability to disable the collector, tune the collection frequency, and set debugging options.
How does Python manage memory?
Python uses a private heap to manage memory. The heap holds all the objects and data structures created by the program. The heap is the memory space where all the objects are stored. The heap is managed by the Python memory manager.
The Python memory manager has different components:
The memory allocator: The memory allocator is responsible for allocating memory for objects and data structures.
The garbage collector: The garbage collector is responsible for reclaiming memory occupied by objects that are no longer in use by the program.
The memory deallocator: The memory deallocator is responsible for deallocating memory when an object is no longer needed.
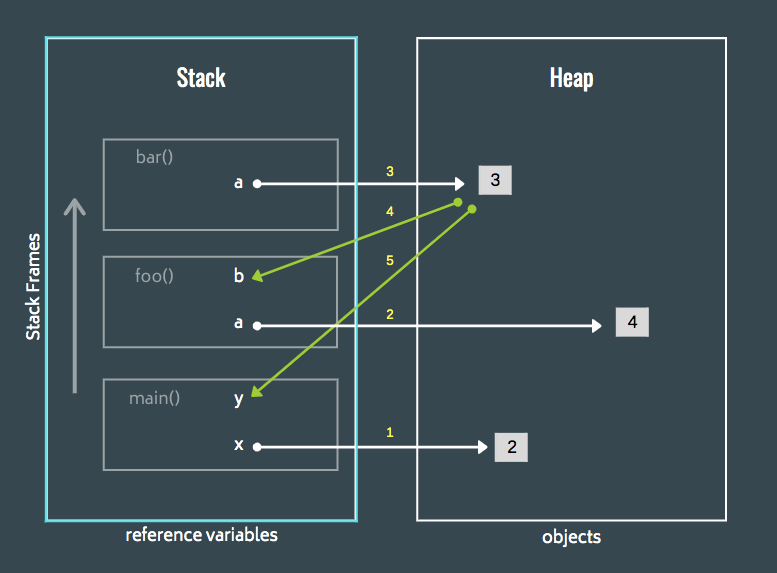
What is garbage collection?
How does garbage collection work in Python?
Python uses a reference counting mechanism to manage memory. Reference counting is a simple and efficient memory management technique. It works by keeping track of the number of references to an object. When an object is created, its reference count is set to one. When an object is referenced by another object, its reference count is incremented. When an object is no longer referenced, its reference count is decremented. When an object’s reference count reaches zero, the object is no longer in use by the program and can be reclaimed by the garbage collector.
Python also uses a cyclic garbage collector to handle reference cycles. A reference cycle occurs when two or more objects reference each other in a circular manner. The cyclic garbage collector is responsible for detecting and breaking reference cycles.
How to disable garbage collection?
You can disable garbage collection in Python using the gc
module. The gc
module provides an interface to the optional garbage collector. You can disable the garbage collector by setting the gc.disable()
function.
Here is an example that demonstrates how to disable garbage collection in Python:
How to enable garbage collection?
You can enable garbage collection in Python using the gc
module. The gc
module provides an interface to the optional garbage collector. You can enable the garbage collector by setting the gc.enable()
function.
Here is an example that demonstrates how to enable garbage collection in Python:
import gc
gc.enable()
How to manually run garbage collection?
You can manually run garbage collection in Python using the gc
module. The gc
module provides an interface to the optional garbage collector. You can manually run garbage collection by setting the gc.collect()
function.
Here is an example that demonstrates how to manually run garbage collection in Python:
import gc
gc.collect()
How to get the garbage collection statistics?
You can get the garbage collection statistics in Python using the gc
module. The gc
module provides an interface to the optional garbage collector. You can get the garbage collection statistics by setting the gc.get_stats()
function.
Here is an example that demonstrates how to get the garbage collection statistics in Python:
import gc
gc.get_stats()
gc.get_stats()
returns a list of dictionaries, where each dictionary contains the following keys:
collections
: The number of garbage collections that have occurred.collected
: The total number of objects collected.uncollectable
: The total number of uncollectable objects.garbage
: The total number of objects that are garbage.