6. Object-Oriented Programming#
Recall from the Introduction that an average piece of software is measured in millions of lines of code.
This code is written by many different people, and it is constantly changing. This is a complex and chaotic situation, and the only way to manage it is to impose structure.
Object Oriented Programming (OOP) is a programming paradigm that aims to organize and structure code in a way that makes that makes large codebases more manageable and scalable.
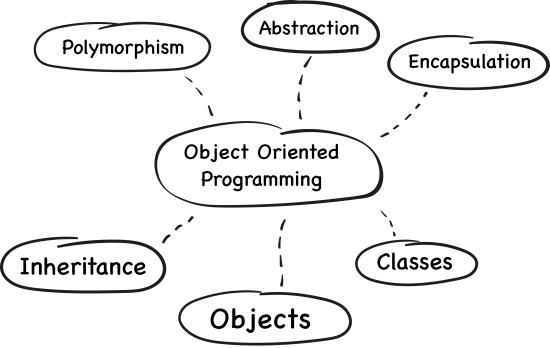
It does this by combining data variables and functions into a single unit. This is a powerful and intuitive way to model the real world in code, and it is the foundation of modern software development.
Combininig data and functions into a single unit reduces complexity by allows for more modular, reusable and readable code.
The core ideas of OOP are given in the figure above. Let’s begin with Abstraction and Encapsulation.
There are two primary abstractions in computer programming that you have already been using:
Data abstractions: A way to refer to, store and manipulate data without having to understand the details of how it is implemented e.g. variables.
Procedural abstractions: A way to refer to, invoke and execute common behaviors without having to understand the details of how they are implemented e.g. functions.
Data Abstractions
The atomic indivisible unit of data in computer programming is called a value. Values are the most basic things that a computer program manipulates or calculates. For example, the number 42
is a value. So is "Hello World!"
.
Variables are the first means of abstraction in computer programming. They allow us to abstract away the details of the value and refer to computations in more general terms.
For instance, \(\pi\) is a value that is approximately 3.14159
. We can refer to this value by the name pi
and use it in calculations without having to remember the value 3.14159
.
Similarly, variables allow us to write equations such as
without having to remember the value of \(\pi\) or concern ourselves with any particular value of \(r\).
Procedural Abstractions
The other primary means of abstraction in programming is the function. A function is a named sequence of statements that performs a computation. When you define a function, you specify the name and the sequence of statements. Later, you can “call” the function by name.
Implementation details of a function are suppressed or abstracted away from the caller. This is called procedural abstraction. The caller of the functions needs to only be concerned with the function’s interface: what input(s) it takes and what output(s) it returns
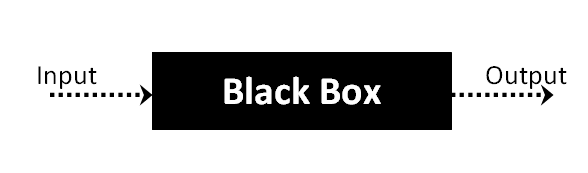
Code: output = blackbox(input)
Math: \( \text{y} = f(\text{x}) \)
Functions are the second means of abstraction in computer programming. They allow us to abstract away the details of the computation and refer to computations in more general terms.
Python comes with many built-in functions such as type(..)
, abs(..)
, round(..)
, help(..)
, sorted()
etc.
Take sorted
for example. We can use the sorted
function to sort a list of numbers without having to understand the details of how the sorting is implemented. Internally, sorted
might be using mergesort or selection sort or quicksort or any other sorting algorithm. In fact, it is using a sorting algorithm called Timsort. We don’t need to know anything about Timsort to use sorted
.
We only need to know the interface of sorted
. The interface of a function is defined by its name, the number of arguments it takes, and the type of its arguments. The interface of sorted
is sorted(iterable, *, key=None, reverse=False) -> list
. This means that sorted
takes an iterable as its first argument and returns a list. The other two arguments are optional.
We can see any function’s interface by calling the help
function with the function as an argument. For example, to read the interface of the abs
function, you can call help(abs)
.
help(abs)
Object-Oriented Programming = Data + Procedural Abstraction
The basic idea of object-oriented programming is to encapsulate data abstractions and procedural abstractions into a single super-abstraction called an Object.


Fig. 6.1 The process of bundling up data and methods associated with that data into a single unit is called Encapsulation.#
In other words, encapsulation is the idea of combining data and the functions that operate on the data into the same single unit. It is a way to hide the implementation details of a class from the user of the class.